How to implement Reset Password in ASP.NET Core Identity with Email? If this is your question you opened up the right post.
What is Reset Password?
If a user forgets the password, the User will input email address of login credentials and clicks on forget password. If the user exists in the database with the provided email, system will send a Reset Password email to the user with a link and link contains the specific token to reset password.
Reset/Forgot Password is the very basic and important feature/functionality of any website or portal. If it comes to ASP.NET Core Identity we are here to guide you. How simply you can implement this functionality into your ASP.NET Core Project. In this tutorial, I’m using the latest .NET Core Version 5 and my project is ASP.NET Core Web API. If you have not configured identity into your project you can follow our tutorial.
Tools:
Let’s get started
This is our API method for token generation and link building. In this method we are taking user’s email as an argument and finding the user with the email. If the user exists in database we will pass the user to the “GeneratePasswordResetTokenAsync(ApplicationUser)” . “GeneratePasswordResetTokenAsync(ApplicationUser)” this method will take the application user as an argument which user wants to reset the password. And generate a token for that specific user. After the Reset password token generation, we will create a link for our next API. That will confirm this forgot/reset password token and create a new password.
public async Task<ResponseViewModel<object>> SendResetPwdLink(string email)
{
try
{
var user = await _userManager.FindByEmailAsync(email);
var token = await _userManager.GeneratePasswordResetTokenAsync(user);
var link = "https://localhost:44379/api/account/confirmresetpassword?";
var buillink = link + "&Id=" + user.Id + "&token=" + token;
var emailtemplate = new EmailTemplate();
emailtemplate.Link = buillink;
emailtemplate.UserId = user.Id;
emailtemplate.EmailType = EmailType.ResetPassword;
var emailsent = _emailService.SendSmtpMail(emailtemplate);
if(emailsent != true)
throw new HttpStatusException(System.Net.HttpStatusCode.InternalServerError, "Email not sent.");
return new ResponseViewModel<object>
{
Status = true,
Message = "Link Sent Succesfully",
StatusCode = System.Net.HttpStatusCode.OK.ToString(),
Data = buillink
};
}
catch (Exception e)
{
throw e;
}
}
After generating the token we are building a link to confirm our generated token. And the link that we are building is for our next API that will confirm our token and update the password. We are also attaching the user Id. And token with the link as a query parameter so we can get the user and token into our confirm reset password API. After creating a proper link we are sending the link to our user email account. If you have not yet implemented an email sending service. Follow these tutorials For Gmail API and SMTP email services. Let’s move forward.
In the below method we are receiving three parameters as arguments. These are the arguments that we have added into our link in the above method. With the id we will find the specific user and pass it forward. To confirm our reset/forgot password token and update the new password. We will use “ResetPasswordAsync(ApplicationUser, string Token, string newPassword)“. It will take three arguments as a parameter Specific Identity user for whom we want to update the password. Reset Password Token and a new password that the user wants to update.
public async Task<ResponseViewModel<object>> ConfirmPwdLink(Guid id, string token, string newpassword)
{
var user = _context.Users.FirstOrDefault(s => s.Id == id);
var result = await _userManager.ResetPasswordAsync(user, token, newpassword);
if (!result.Succeeded)
{
return new ResponseViewModel<object>
{
Status = false,
Message = "Invalid Request",
StatusCode = System.Net.HttpStatusCode.UnprocessableEntity.ToString(),
};
}
else
{
return new ResponseViewModel<object>
{
Status = true,
Message = "Your Password has been succesfully updated",
StatusCode = System.Net.HttpStatusCode.OK.ToString()
};
}
}
You will receive an email like this,
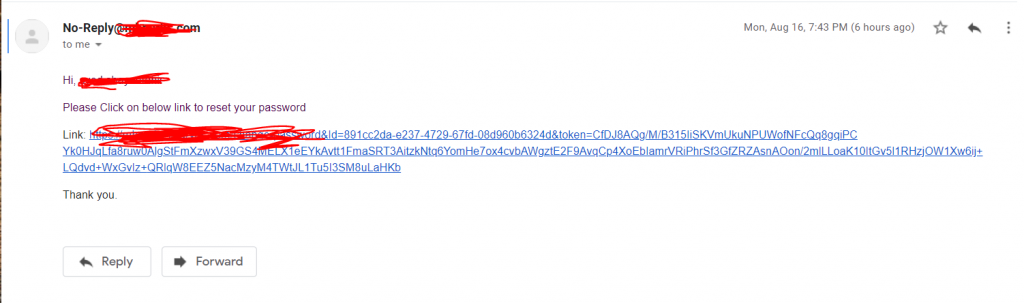
Copy this link and paste it as it is in postman so you can add a new password to it. It will look like this and return the response true if our token will be successfully confirmed.
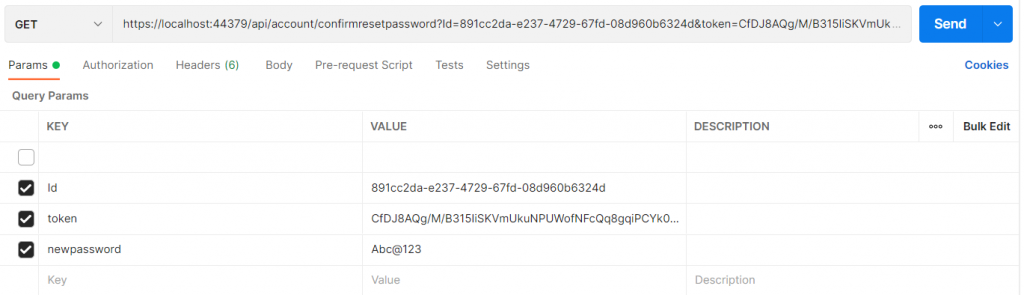
I have also written a complete tutorial on email Confirmation with ASP.NET Core Identity.
Customizing the Password Reset with Email Feature in ASP.NET Core Identity
ASP.NET Core Identity offers several customization options for the password reset with email feature. These customization options allow developers to tailor the feature to meet their specific project requirements.
One customization option available in ASP.NET Core Identity is the ability to specify the format of the email sent to the user when a password reset request is initiated. The default email template provided by ASP.NET Core Identity may not be suitable for all projects, so developers can modify the email template to include project-specific information, such as branding or custom messages.
To modify the email template, developers can create a new email template in HTML format and include placeholders for the dynamic content. The dynamic content includes information such as the user’s email address, a link to reset the password, and any custom messages. Developers can then use the email service provider’s API to send the email with the dynamic content.
Another customization option available in ASP.NET Core Identity is the ability to specify the expiration time for the password reset link. By default, the link expires after 24 hours. However, for projects that require tighter security or faster turnaround time, developers can change the expiration time to a shorter or longer duration.
To change the expiration time, developers can modify the token provider settings in the ASP.NET Core Identity configuration. The token provider generates the password reset token, and developers can set the expiration time for the token. They can also configure the token provider to generate a new token each time a password reset request is initiated, which provides additional security by preventing the reuse of expired tokens.
Finally, developers can customize the error messages displayed to the user during the password reset process. By default, ASP.NET Core Identity provides generic error messages that may not be informative or user-friendly. Developers can modify the error messages to provide more specific information or to match the project’s tone and voice.
To customize the error messages, developers can override the default messages in the ASP.NET Core Identity configuration. They can also implement custom logic to handle specific error conditions, such as invalid email addresses or expired password reset links.
Testing and Deployment of the Password Reset with Email Feature
Once the password reset with email feature has been implemented and customized, it is important to thoroughly test it before deploying it to production. This ensures that the feature works as expected and that any issues are identified and resolved before they impact users.
To test the feature, developers can create test accounts with different email addresses and initiate password reset requests. They can verify that the email is sent with the correct content and that the password reset link works as expected. Developers can also test the expiration time of the link to ensure that it expires after the configured duration.
In addition to testing the functionality of the password reset with email feature, developers should also test its security. They can do this by attempting to use expired or invalid password reset links to reset passwords and verifying that they are rejected. Developers can also test the feature’s resilience to attacks, such as brute force attacks or injection attacks, by using security testing tools.
Once testing is complete, the password reset with email feature can be deployed to production. Before deployment, developers should ensure that the email service provider is configured correctly and that any necessary credentials or API keys are set up. They should also ensure that the feature is integrated with any existing authentication and authorization mechanisms and that it does not introduce any security vulnerabilities.
After deployment, developers should continue to monitor the feature for issues or vulnerabilities and make any necessary updates or changes. This ensures that the feature continues to provide a secure and user-friendly password reset experience for users.
Conclusion
You can configure Reset/forgot password API by following these simple steps that we have mentioned above. if you face any issue or problem while configuring this method with your ASP.NET Core project feel free to comment below. We will try to respond ASAP.