We can handle our errors/exceptions by simply using try-catch blocks. But What if we have to throw custom exceptions on some error. To achieve this custom exception throw approach follow the below-mentioned tutorial.
Custom exception handling and throwing custom exceptions is very important to maintain a standard Web Application. It will create a difference and your code will look more clean and standard. In this post, we are going to learn that how can we throw custom exceptions in our ASP.NET Core Web API project.
Tools:
Windows OS
Let’s get started:
If we have to throw a custom exception on some error, Like we have to throw a custom exception in response to signup API if we do not receive a strong password according to our requirement. To implement this we have to create a class that we can use to throw our exception response.
public class MyExceptionResponse { public string Type { get; set; } public string Message { get; set; } public string StackTrace { get; set; } public bool Status { get; set; } public int StatusCode { get; set; } public MyExceptionResponse(Exception ex) { Type = ex.GetType().Name; Message = ex.Message; Status = false; StackTrace = ex.ToString(); StatusCode = 500; if (ex is HttpStatusException httpException) { //this.StatusCode = httpException.Status.ToString(); this.StatusCode = (int)httpException.Status; } } public class HttpStatusException : Exception { public HttpStatusCode Status { get; set; } public HttpStatusException(HttpStatusCode code, string msg) : base(msg) { this.Status = code; } } }
This is the class that we will use to throw our all exceptions globally. In this class, we can see we have another class that we will use to throw custom exceptions on our custom login and wherever we want to throw a custom error in response. We are deriving this class from our main “Exception” class. Now we have to create a controller that will convert our error exceptions to above mentioned
“MyExceptionResponse.cs” class.
[ApiController] [ApiExplorerSettings(IgnoreApi = true)] public class ExceptionController : ControllerBase { [Route("exception")] public MyExceptionResponse Error() { var context = HttpContext.Features.Get<IExceptionHandlerFeature>(); var exception = context?.Error; // Your exception var code = 500; // Internal Server Error by default if (exception is HttpStatusException httpException) { code = (int)httpException.Status; } Response.StatusCode = code; return new MyExceptionResponse(exception); // Your error model } }
Add this into your “startup.cs” at the top of the middleware list.
app.UseExceptionHandler("/exception");
After adding this controller into our “Startup.cs” as a middleware so whenever any exception will occur in the project. This exception controller will be called automatically from the middleware pipeline.
Now let’s see how we can throw and handle custom exceptions. This is our custom logic where we want to handle and throw a custom exception.
var passwordValidator = new PasswordValidator<ApplicationUser>(); var valid = await passwordValidator.ValidateAsync(_userManager, null, user.Password); if(!valid.Succeeded) throw new HttpStatusException(System.Net.HttpStatusCode.UnprocessableEntity, valid.Errors.FirstOrDefault().ToString());
You can also send a custom message in the exception by adding a string into your exception like this.
var passwordValidator = new PasswordValidator<ApplicationUser>(); var valid = await passwordValidator.ValidateAsync(_userManager, null, user.Password); if(!valid.Succeeded) throw new HttpStatusException(System.Net.HttpStatusCode.UnprocessableEntity, "Passwords must have at least one uppercase ('A'-'Z'), one digit ('0'-'9') and one non alphanumeric character.")
So if we have a list of errors and want to send all errors to the user interface we will add a custom string summing up all the errors.
Now let our error/exception on this logic.
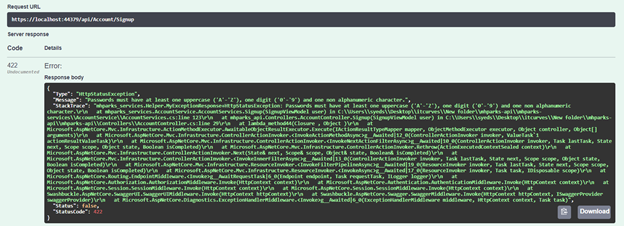
You can see the type, our custom message, status, and status code as well.
Overview of Exceptions in ASP.NET Core Web API
When building an application in ASP.NET Core Web API, it is important to understand what exceptions are and how they are handled. In simple terms, an exception is an event that occurs during the execution of a program that disrupts its normal flow.
In ASP.NET Core Web API, there are different types of exceptions that can occur. Some common types include:
- System.ArgumentNullException: This exception is thrown when a method or property is called with a null argument.
- System.FormatException: This exception is thrown when a string cannot be parsed as the expected type.
- System.InvalidOperationException: This exception is thrown when a method call is invalid for the object’s current state.
When an exception is not handled, it can cause your application to crash or behave in unexpected ways. By default, ASP.NET Core Web API provides a global exception handling mechanism that catches unhandled exceptions and returns an error response to the client.
However, it is often useful to create custom exceptions to provide more meaningful error messages and to handle specific scenarios in your application. Custom exceptions can be created by deriving from the base Exception class or one of its subclasses.
Throwing Custom Exceptions in ASP.NET Core Web API
Throwing custom exceptions in ASP.NET Core Web API allows you to provide more descriptive error messages to clients and handle specific error scenarios. In this section, we will explore how to throw custom exceptions in your API code.
A. How to throw custom exceptions in ASP.NET Core Web API To throw a custom exception in ASP.NET Core Web API, you first need to define the exception. This is usually done by creating a new class that inherits from the Exception class or one of its subclasses.
Once you have defined your custom exception class, you can throw it in your API code using the throw keyword. For example, if you have a custom exception called InvalidRequestException, you could throw it like this:
throw new InvalidRequestException(“The request was invalid.”);
Catching and handling custom exceptions in ASP.NET Core Web API
When a custom exception is thrown in your API code, it is important to catch and handle it appropriately. This can be done using try-catch blocks.
In ASP.NET Core Web API, you can catch and handle exceptions at different levels of the application. For example, you can catch exceptions at the controller level, or you can catch them globally using middleware.
Here’s an example of catching a custom exception in a controller:
[HttpPost]
public async Task CreateProduct(Product product)
{
if (product == null)
{
throw new InvalidRequestException(“The product object was null.”);
}
// Other code here...
return Ok();
}
[HttpGet(“{id}”)]
public async Task GetProduct(int id)
{
var product = await _productService.GetProductByIdAsync(id);
if (product == null)
{
throw new ProductNotFoundException($"The product with ID {id} was not found.");
}
// Other code here...
return Ok(product);
}
// Exception filter for catching custom exceptions
[ApiController]
[Route(“api/[controller]”)]
[TypeFilter(typeof(CustomExceptionFilterAttribute))]
public class ProductsController : ControllerBase
{
private readonly IProductService _productService;
public ProductsController(IProductService productService)
{
_productService = productService;
}
}
In this example, we are throwing custom exceptions in the CreateProduct and GetProduct actions. We are also using an exception filter to catch any custom exceptions thrown by our actions and handle them appropriately.
C. Examples of throwing and handling custom exceptions in ASP.NET Core Web API Here are a few more examples of throwing and handling custom exceptions in ASP.NET Core Web API:
- If an API client submits an invalid date format in a request, you could throw a custom exception called InvalidDateFormatException and provide a helpful error message.
- If your API code encounters an unexpected error, you could throw a custom exception called InternalServerErrorException and log the error for troubleshooting.
- If a request exceeds a rate limit, you could throw a custom exception called RateLimitExceededException and return a response indicating that the rate limit has been exceeded.
Best Practices for Using Custom Exceptions in ASP.NET Core Web API
While custom exceptions can be useful in ASP.NET Core Web API, it is important to use them wisely to avoid causing confusion or unnecessary complexity. In this section, we will discuss some best practices for using custom exceptions in your API code.
Guidelines for using custom exceptions in ASP.NET Core Web API
When creating and using custom exceptions in ASP.NET Core Web API, here are some guidelines to keep in mind:
- Keep custom exceptions specific: Custom exceptions should be specific to the error scenario they represent. Avoid creating generic exceptions that could apply to multiple scenarios, as this can lead to confusion and make it harder to handle errors correctly.
- Provide helpful error messages: When throwing custom exceptions, be sure to provide helpful error messages that provide insight into what went wrong and how to resolve the issue. Avoid cryptic error messages that do not provide any context or guidance.
- Use HTTP status codes appropriately: When throwing custom exceptions, be sure to use the appropriate HTTP status code for the error scenario. This can help clients understand the severity of the error and how to respond to it.
- Be consistent: When creating and using custom exceptions, be consistent in your naming conventions and error messages. This can make it easier to maintain and update your API code over time.
Common mistakes to avoid when using custom exceptions
Here are some common mistakes to avoid when using custom exceptions in ASP.NET Core Web API:
- Creating too many custom exceptions: Avoid creating too many custom exceptions, as this can lead to unnecessary complexity and make it harder to handle errors correctly. Instead, focus on creating a few specific exceptions that cover the most common error scenarios.
- Using custom exceptions for expected errors: Custom exceptions should be reserved for unexpected errors or exceptional situations. Avoid using custom exceptions for errors that are expected as part of normal operation.
- Throwing exceptions too early: Avoid throwing exceptions too early in your API code, as this can make it harder to handle errors correctly. Instead, try to handle errors as early as possible without throwing exceptions.
Tips for effective use of custom exceptions
Here are some tips for effectively using custom exceptions in ASP.NET Core Web API:
- Use logging: When throwing custom exceptions, be sure to log the error details for troubleshooting and analysis.
- Test your code: Before deploying your API code, be sure to thoroughly test your custom exceptions to ensure they are working as expected.
- Use exception filters: Consider using exception filters to catch and handle custom exceptions globally, rather than in each action.
- Document your exceptions: Be sure to document your custom exceptions in your API documentation, including their expected error scenarios, error messages, and HTTP status codes.
Conclusion
This is a standard and quality way to handle custom exceptions in ASP.NET Core Web API Project. Try this tutorial and if you do not understand anything or face any issue while applying this method. Do not hesitate to comment below. MYCODEBIT team will try to respond ASAP.