In one of the previous tutorials, we have created our project and configured Identity using Entity Framework Core. Now we will create RESTful APIs to perform Login and Registration Using Identity In ASP.NET Core.
If you have not implemented Identity Read my previous tutorial to implement it by clicking on the above-attached link. It seems difficult but believe me it’s pretty simple. Just follow my instructions and practice the code with me.
First of all, we have to write services to perform signup/registration, login/sign in, and logout then we will call these services from our controller.
I have added a class into my model section into the “ViewModel” Section to return and handle responses from my services.
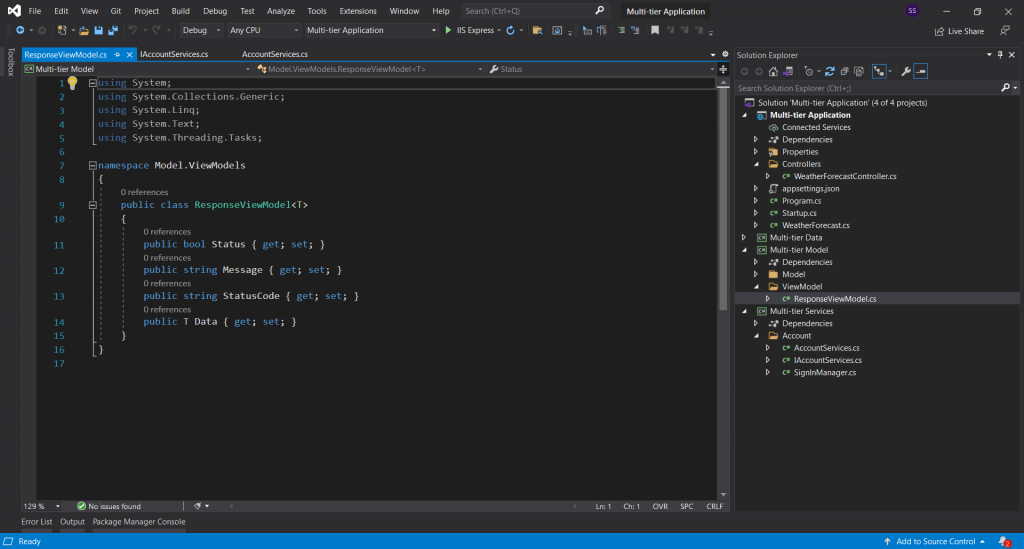
And here is the “ResponseViewModel.cs” class.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace Model.ViewModels
{
public class ResponseViewModel<T>
{
public bool Status { get; set; }
public string Message { get; set; }
public string StatusCode { get; set; }
public T Data { get; set; }
}
}
Add folder into your services section and name it “AccountService”.
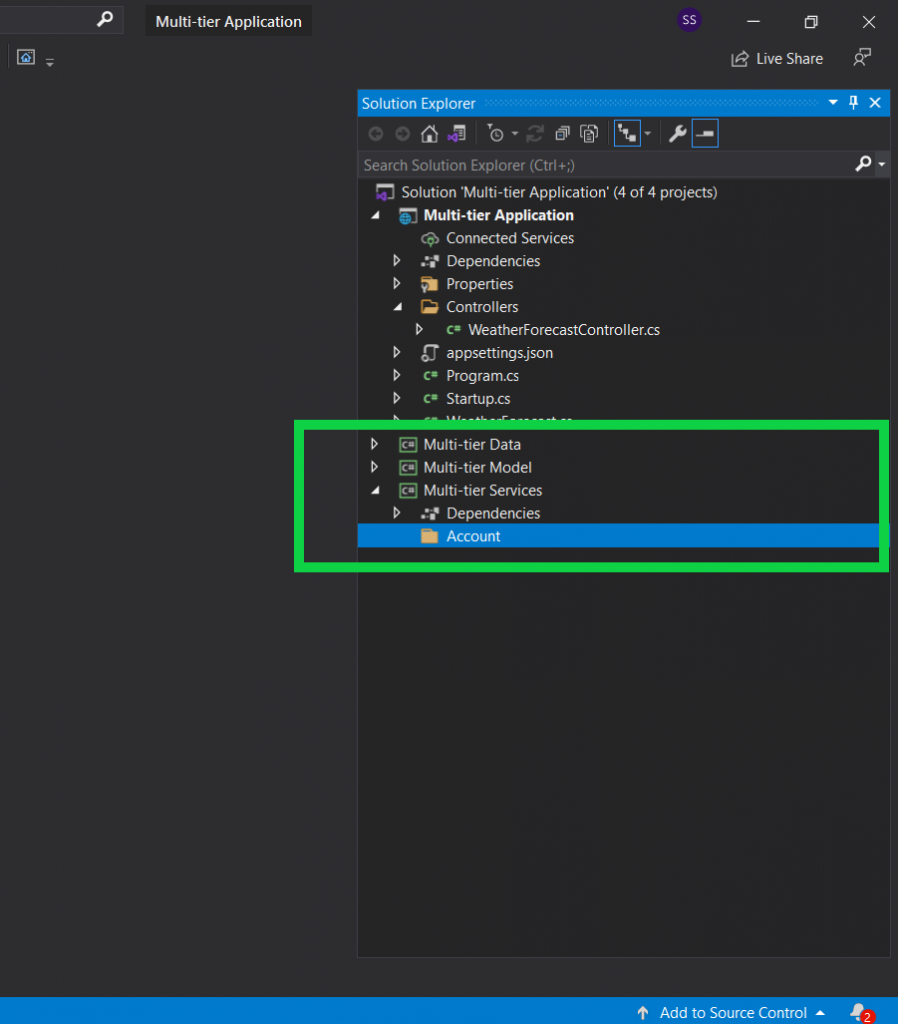
Now add class into your “AccountService” folder and name it “AccountServices.cs” and drive it from “IAccountServices” to create our method signatures. And we will implement these methods into our “AccountServices.cs” class.
First Create signatures of your methods into your “IAccountServices.cs”
using Microsoft.AspNetCore.Mvc;
using Multi_tier_Model.Model;
using System.Threading.Tasks;
namespace Multi_tier_Services.Account
{
public interface IAccountServices
{
public Task<ResponseViewModel<object>> Post(ApplicationUser user);
public Task<ResponseViewModel<object>> LogIn(ApplicationUser user);
public Task<ResponseViewModel<object>> LogOut(string username);
}
}
After the signature of the methods implement these methods in the “AccountClass.cs”
using Microsoft.AspNetCore.Identity;
using Model.ViewModels;
using Multi_tier_Data.Data;
using Multi_tier_Model.Model;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace Multi_tier_Services.Account
{
public class AccountServices: IAccountServices
{
private readonly ApplicationDbContext _context;
private readonly UserManager<ApplicationUser> _userManager;
private readonly SignInManager<ApplicationUser> _signInManager;
public AccountServices(ApplicationDbContext context,
UserManager<ApplicationUser> userManager,
SignInManager<ApplicationUser>signInManager)
{
_context = context;
_userManager = userManager;
_signInManager = signInManager;
}
public async Task<ResponseViewModel<object>>Post(ApplicationUser data)
{
try
{
await _userManager.CreateAsync(data);
return new ResponseViewModel<object>
{
Status = true,
Message = "Data Added Succesfully",
};
}
catch (Exception e)
{
return new ResponseViewModel<object>
{
Status = false,
Message = e.Message,
};
}
}
public async Task<ResponseViewModel<object>> LogIn(ApplicationUser data)
{
try
{
var user = await _userManager.FindByNameAsync(data.UserName);
if(user == null)
{
return new ResponseViewModel<object>
{
Status = false,
Message = "Invalid UserName",
};
}
var result = _signInManager.PasswordSignInAsync(user, user.PasswordHash, false, false);
if (result.IsCompleted == true)
{
return new ResponseViewModel<object>
{
Status = true,
Message = "SignIn Succesfully",
};
}
return new ResponseViewModel<object>
{
Status = false,
Message = "Wrong Password",
};
}
catch (Exception e)
{
return new ResponseViewModel<object>
{
Status = false,
Message = e.Message,
};
}
}
public async Task<ResponseViewModel<object>> LogOut(string username)
{
var user = await _userManager.FindByNameAsync(username);
await _userManager.UpdateSecurityStampAsync(user);
return new ResponseViewModel<object>()
{
Status = true,
Message = "User Logged Out",
StatusCode = System.Net.HttpStatusCode.OK.ToString()
};
}
}
}
To logout, use add this code into your "startup.cs" class
//to logout User
services.Configure<SecurityStampValidatorOptions>(options =>
{
// enables immediate logout, after updating the user's stat.
options.ValidationInterval = TimeSpan.Zero;
});
Do not forget to configure your “AccountServices” into your startup class.
services.AddTransient<IAccountServices, AccountServices>();
Now we have implemented our business logic in service now we have to make calls to these services through our APIs.
Create an API controller “AccountController.cs” into your main API project under the Controller folder.
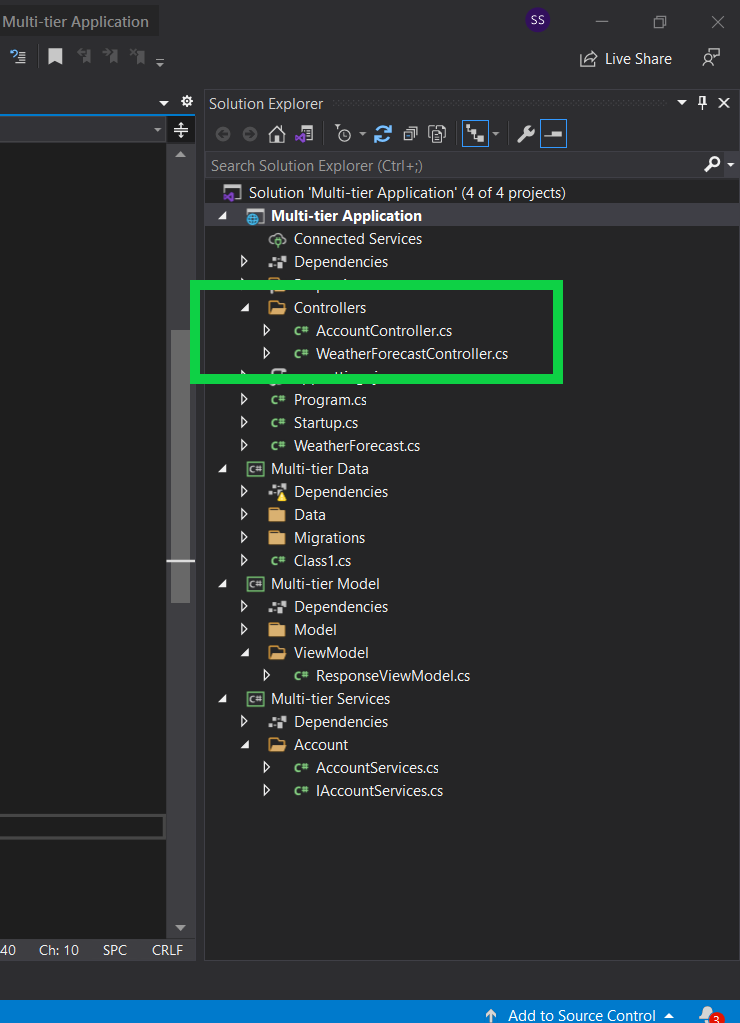
Here is the “AccountController.cs” Code
using Microsoft.AspNetCore.Http;
using Microsoft.AspNetCore.Mvc;
using Model.ViewModels;
using Multi_tier_Model.Model;
using Multi_tier_Services.Account;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
namespace _3tier.Controllers
{
[Route("api/[controller]")]
[ApiController]
public class AccountController : ControllerBase
{
private readonly IAccountServices _services;
public AccountController(IAccountServices services)
{
_services = services;
}
[HttpPost]
public Task<ResponseViewModel<object>> Post(ApplicationUser data)
{
return _services.Post(data);
}
[HttpPost("Login")]
public Task<ResponseViewModel<object>> LogIn(ApplicationUser data)
{
return _services.LogIn(data);
}
[HttpPost("Logout")]
public Task<ResponseViewModel<object>> Logout(string username)
{
return _services.LogOut(username);
}
}
}
Here is how my project solution looks like.
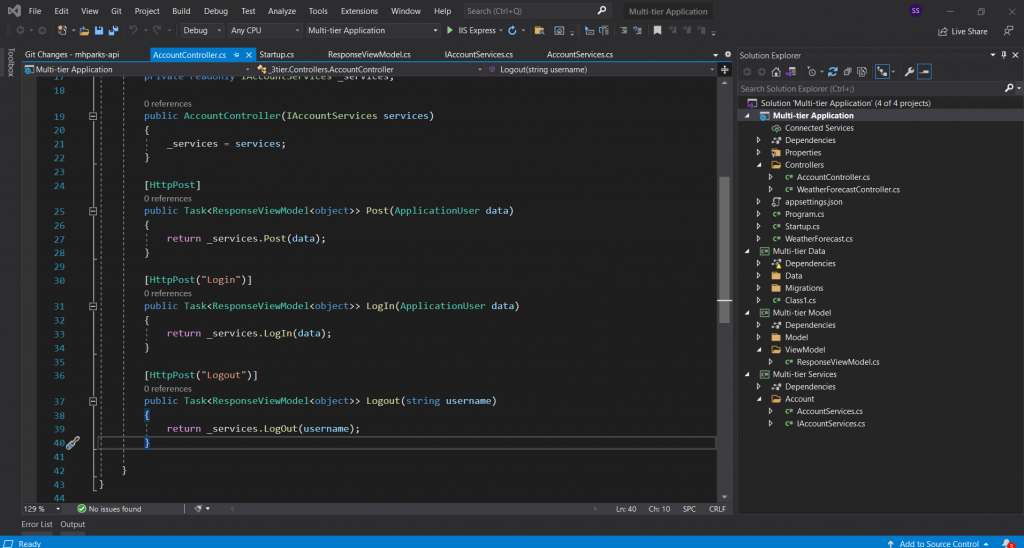
run the project and you will get the screen something like this.
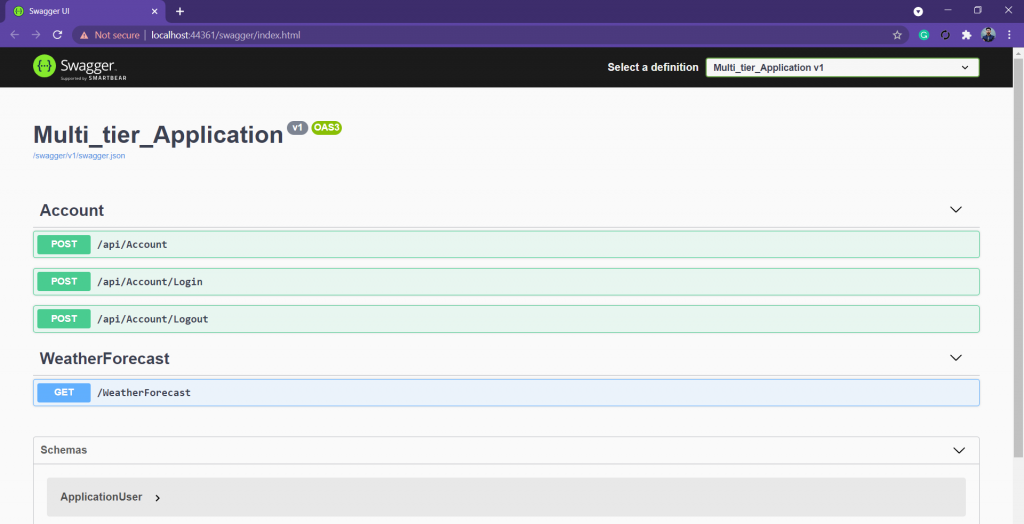
Summery
In this article, we have performed registration And Login Functionality In ASP.NET Core 5 Web API Application Using 3-tier Identity. If you face any problem or have any query in your mind do not hesitate to leave a comment below or contact us.
Soon we will write an article about “Asp.net core mvc login and registration using identity” Stay Tuned!