By reading this title some beginners and new to programming peeps will be amazed that how can we pass a JSON object as a query parameter in “Get” type API using ASP.NET Core.
In this post, we will discuss and learn that how can we perform this task in our ASP.NET Core Web API project. It is very simple and easy.
Tools:
Windows OS
Let’s get started:
We will perform this task in the ASP.NET Core Web API project. If you want to create ASP.NET Core N-tier Application you can set it up by following this LINK.
First of all, we have to create a Model that we are going to receive in the “GET” API as an object. Let’s create a class we are going to name it “FilterViewModel.cs”
public class FilterViewModel { public int PageNo { get; set; } public int PageSize { get; set; } public string Query { get; set; } public string SortBy { get; set; } public bool? SortDesc { get; set; } public bool? Status { get; set; } }
This is the object that we will receive as a query parameter in our API.
Now we will create two classes that will be extended by “IModelBinderProvider”.
public class FilterModelBinder : IModelBinder { public Task BindModelAsync(ModelBindingContext bindingContext) { var jsonString = bindingContext.ActionContext.HttpContext.Request.Query["Filter"]; FilterViewModel result = JsonConvert.DeserializeObject<FilterViewModel>(jsonString); bindingContext.Result = ModelBindingResult.Success(result); return Task.CompletedTask; } } public class FilterModelBinderProvider : IModelBinderProvider { public IModelBinder GetBinder(ModelBinderProviderContext context) { if (context.Metadata.ModelType == typeof(FilterViewModel)) return new FilterModelBinder(); return null; } }
This “FilterModelBinderProvider” class will check the query parameter and if it’s “FilterViewModel.cs” type it will return a response “new FilterModelBinder()” and in this ”FilterModelBinder” class we have implemented a method that will deserialize the JSON Object in our “FilterViewModel”. Please note that we clearly mentioned the name of our object while we are binding query requests.
bindingContext.ActionContext.HttpContext.Request.Query["Filter"];
Please make sure that the object you are sending in the query parameter does have the same name as you have described over here.
Now we have implemented our logic in this class. We also have to configure this “ModelBinderProvider” in our “Startup.cs” class.
services.AddControllers(options => { options.ModelBinderProviders.Insert(0, new FilterModelBinderProvider()); options.Filters.Add(typeof(Authorization)); }).AddNewtonsoftJson(opts => { opts.SerializerSettings.ContractResolver = new DefaultContractResolver(); opts.SerializerSettings.ReferenceLoopHandling = Newtonsoft.Json.ReferenceLoopHandling.Ignore; }).AddJsonOptions(opt => opt.JsonSerializerOptions.PropertyNamingPolicy = null); }
Here we have configured our “ModelBinderProvider”. Now if any of our GET API requests will ever have an object with the name of “filter” It will be deserialized in the “FilterViewModel” type.
Note: You can not call this API from swagger this API will only be called by your front-end frameworks like VueJS, ReactJS, Angular, or Postman.
Your API will look like this.
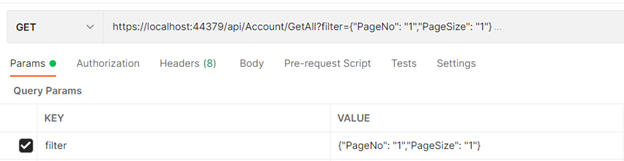
Basic concept of query parameters in ASP.NET Core
In ASP.NET Core, query parameters are a way to include additional information in the URL when making a GET request to an API. Query parameters consist of a key-value pair that are appended to the end of a URL after a question mark (?).
For example, suppose we have an API endpoint that returns a list of books, and we want to filter the results by the book’s title. We can pass the title as a query parameter like this:
GET /api/books?title=The%20Great%20Gatsby
In this example, title
is the key, and The Great Gatsby
is the value. The %20
represents a space character in the URL encoding.
To read the query parameters in ASP.NET Core, we can use the HttpContext.Request.Query
property. This property provides access to a Dictionary<string, StringValues>
object, where the key is the query parameter name, and the value is a collection of string values associated with that key.
For example, to get the value of the title
query parameter in our example above, we can use the following code:
string title = HttpContext.Request.Query[“title”];
If the query parameter is not present in the URL, the Query
property will return an empty collection.
It’s important to note that query parameters are limited in size and should not be used to transmit large amounts of data. In cases where large amounts of data need to be transmitted, consider using the request body instead.
Understanding the basic concept of query parameters in ASP.NET Core is crucial for building robust and flexible APIs. In the next section, we will explore how to pass simple data types as query parameters in ASP.NET Core.
How to pass simple data types as query parameters
Passing simple data types as query parameters in ASP.NET Core is a straightforward process. Simple data types include string, integer, boolean, and other primitive types.
To pass a simple data type as a query parameter, we need to append the key-value pair to the URL when making a GET request to the API endpoint. The key is the name of the query parameter, and the value is the data we want to pass.
For example, to pass a string value as a query parameter to an API endpoint that retrieves customer data, we can do the following:
GET /api/customers?name=John
In this example, name
is the key, and John
is the value. The API endpoint can retrieve the name
value using the HttpContext.Request.Query
property, as shown in the previous section.
Similarly, to pass an integer value as a query parameter, we can do the following:
GET /api/products?price=50
In this example, price
is the key, and 50
is the value. Again, the API endpoint can retrieve the price
value using the HttpContext.Request.Query
property.
We can also pass boolean values as query parameters by using the true
or false
keyword. For example:
GET /api/products?in_stock=true
In this example, in_stock
is the key, and true
is the value.
It’s important to note that when passing string values as query parameters, we need to URL encode the value to ensure it is properly transmitted in the URL. We can use the HttpUtility.UrlEncode
method in the System.Web
namespace to encode the value.
How to pass complex objects as query parameters
In addition to simple data types, we can also pass complex objects as query parameters in ASP.NET Core. Complex objects are objects that contain multiple properties and values.
To pass a complex object as a query parameter, we need to serialize the object into a string representation and append it to the URL as a query parameter. We can use the JsonConvert.SerializeObject
method in the Newtonsoft.Json
namespace to serialize the object into a JSON string.
For example, suppose we have a Product
class with the following properties:
public class Product
{
public int Id { get; set; }
public string Name { get; set; }
public decimal Price { get; set; }
public bool InStock { get; set; }
}
To pass a Product
object as a query parameter to an API endpoint that retrieves products, we can do the following:
Product product = new Product { Id = 1, Name = “Widget”, Price = 50.00m, InStock = true };
string serializedProduct = JsonConvert.SerializeObject(product);
string encodedProduct = HttpUtility.UrlEncode(serializedProduct);
string url = $”/api/products?product={encodedProduct}”;
In this example, we first create a Product
object and serialize it into a JSON string using the JsonConvert.SerializeObject
method. We then URL encode the JSON string using the HttpUtility.UrlEncode
method to ensure it is properly transmitted in the URL. Finally, we append the encoded string to the URL as a query parameter.
To retrieve the Product
object in the API endpoint, we can deserialize the JSON string back into a Product
object using the JsonConvert.DeserializeObject
method in the Newtonsoft.Json
namespace.
It’s important to note that passing complex objects as query parameters can lead to long and unwieldy URLs, which can cause issues with URL length limitations in some browsers and servers. Additionally, passing sensitive information as query parameters is not recommended, as the information is visible in the URL.
Common pitfalls and best practices
While passing objects as query parameters in ASP.NET Core can be a useful feature, there are some common pitfalls and best practices to keep in mind.
- URL length limitations: As mentioned in the previous section, passing large objects as query parameters can lead to long and unwieldy URLs, which can cause issues with URL length limitations in some browsers and servers. It’s important to keep the size of query parameters to a minimum and consider using the request body instead for large amounts of data.
- Security risks: Passing sensitive information as query parameters is not recommended, as the information is visible in the URL. Consider using the request body or headers to transmit sensitive information instead.
- Naming conventions: When naming query parameters, it’s important to follow naming conventions and use descriptive names that accurately reflect the data being passed. This helps to avoid confusion and makes the API easier to understand and use.
- Data validation: Always validate the data being passed as query parameters to ensure it is in the correct format and meets any applicable constraints. This helps to prevent unexpected errors and improve the overall reliability of the API.
- Error handling: When errors occur while processing query parameters, it’s important to handle them gracefully and provide informative error messages to the client. This helps to improve the user experience and make the API easier to debug and troubleshoot.
By following these best practices, we can ensure that our APIs are robust, secure, and easy to use. Understanding the common pitfalls associated with passing objects as query parameters is crucial for building high-quality APIs.
Conclusion
Try this tutorial and if you do not understand anything or face any issue while applying this method. Do not hesitate to comment below. Team MYCODEBIT will try to respond ASAP.