In this article, we will try to cover what is gRPC and How to create gRPC application in ASP.NET Core and How to send request from client to gRPC Application in ASP.NET Core. Before getting into gRPC let’s discuss what is RPC.
What is RPC?
Remote Procedure Call (RPC) is a protocol that one program can use to send or receive request from a program located in another computer on a network without understanding the network’s details.
What is gRPC?
gRPC is open-source, schema-first Remote Procedure Call framwork that was created by Google and it takes benifits of advance protocols to transport binary messages like HTTP/2. Protocol bufer is a binary serialization protocol from Google that is used to serialize and deserialize messages in gRPC.
gRPC is supported by numerious programming languages including C++, C#, Java, TypeScript/JavaScript, Go, Python, Ruby and so on.It has become more popular for building distributed systems. gRPC was initially introduced in .NET Core 3.1.
Advantages of gRPC?
Lightweight
Quick Response
Bi-direcctional streaming
Binary Serialization
Communication Patterens of gRPC:
Multiple Communication patterens are supported by gRPC.
Unary RPC: The client sends the single request to the server and server send back a singe response.
Server Streaming RPC: The client sends the single reguest to the server and server sends back a stream of reponses.
Client Streaming RPC: The Client sends a streams of requests to the server and server sends back a single response.
Bi-Directional Straming RPC: The Client sends streams of requests to the server and server sends back a stream of reponses.
How to Create gRPC Application in ASP.NET Core:
Let’s start practicle. We are using Visual Studio 2022 to create gRPC Application in ASP.NET Core 3.1. For this tutorial you should have Visual studio if you don’t have you can download it from here.
1: Open Visual Studio
2: Click on Create Project
3: Search “gRPC” and select project, Click Next
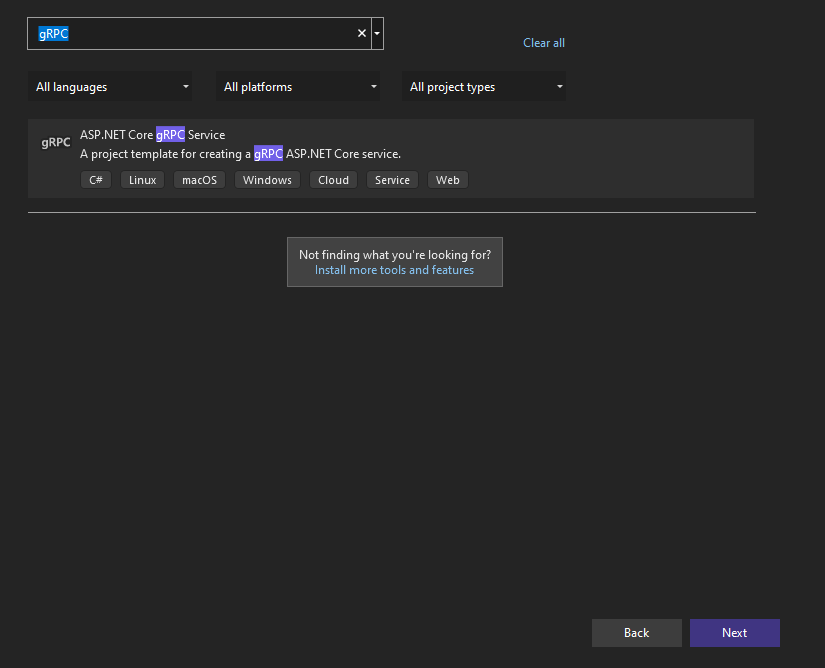
4: Give name to your project and select a location to save project, Click on Next.
5: Select Framework “.Net Core 3.1” and click on create.
6: After all steps you can see the files in solution explorer.
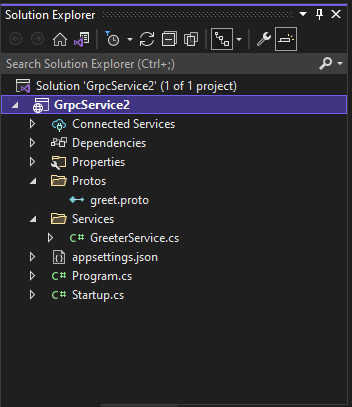
Let’s understand these files first and then we will move forward.
You can see the Protos and Services folder in the above picture. These two are the main solutions of gRPC. In Protos folder we have .proto files that contains the message contracts and services have the all the logics and services.
If we open this greet.proto file, we will have have this code on our screen.
syntax = "proto3"; option csharp_namespace = "GrpcService2"; package greet; // The greeting service definition. service Greeter { // Sends a greeting rpc SayHello (HelloRequest) returns (HelloReply); } // The request message containing the user's name. message HelloRequest { string name = 1; } // The response message containing the greetings. message HelloReply { string message = 1; }
In the above code snippet, Greeter is the name of service, HelloRequest and HelloReply are message contracts. SayHello accepts HelloRequest as a message and returns response as a HelloReply.
Now let’s see the services (.cs) files.
public class GreeterService : Greeter.GreeterBase { private readonly ILogger<GreeterService> _logger; public GreeterService(ILogger<GreeterService> logger) { _logger = logger; } public override Task<HelloReply> SayHello(HelloRequest request, ServerCallContext context) { return Task.FromResult(new HelloReply { Message = "Hello " + request.Name }); } }
We can see the class name or service name as GreeterService that was discribed in .porto file and the GreeterService is derived from Greeter.GreeterBase Class.
If you open “Startup.cs” file you can see this code clearly that is used to configure gRPC services in ASP.NET Core Application.
public void ConfigureServices(IServiceCollection services) { services.AddGrpc(); }
We can also see the MapGrpcService() in the pipline of middlewares that is used add to add pipeline of gRPC service in Middleware.
public void Configure(IApplicationBuilder app, IWebHostEnvironment env) { if (env.IsDevelopment()) { app.UseDeveloperExceptionPage(); } app.UseRouting(); app.UseEndpoints(endpoints => { endpoints.MapGrpcService<GreeterService>(); endpoints.MapGet("/", async context => { await context.Response.WriteAsync("Communication with gRPC endpoints must be made through a gRPC client. To learn how to create a client, visit: https://go.microsoft.com/fwlink/?linkid=2086909"); }); }); }
How to send request from client to gRPC Application in ASP.NET Core:
Now let’s create a client and send request to this gRPC ASP.NET Core service. To create a client we have create a console application in .NET Core. Follow the steps
1: Open Visual studio
2: Click on Create Project
3: select Console App in .NET Core and click on next.
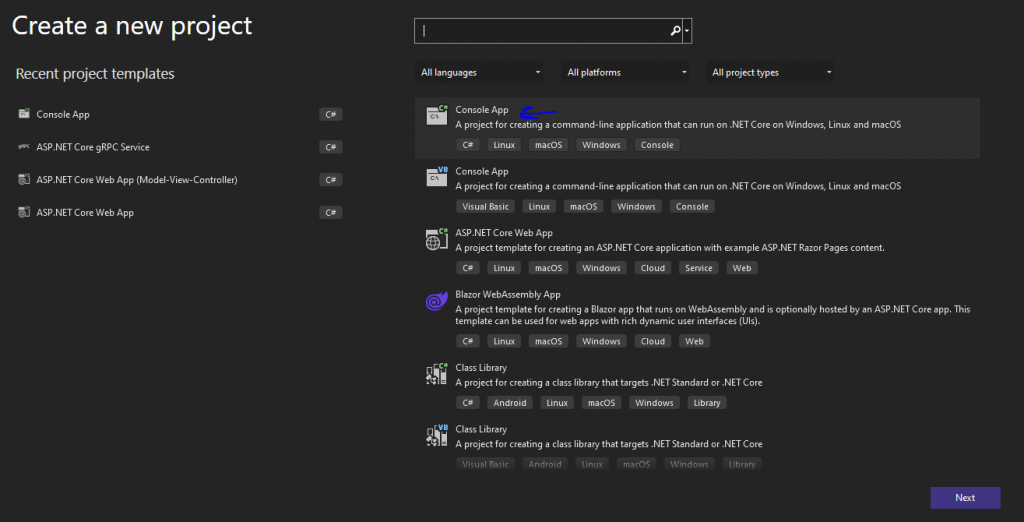
4: Select location and give name to your client project and click on next.
5: Select .NET Core 3.1 as a Framework version
Now you have created your client succesfully now we have to send message to our gRPC Service from our this client. Past this code as you main program in you client console application.
static async System.Threading.Tasks.Task Main(string[] args) { var httpHandler = new HttpClientHandler(); // Return `true` to allow certificates that are untrusted/invalid httpHandler.ServerCertificateCustomValidationCallback = HttpClientHandler.DangerousAcceptAnyServerCertificateValidator; var channel = GrpcChannel.ForAddress("https://localhost:5001", new GrpcChannelOptions { HttpHandler = httpHandler }); var client = new Greeter.GreeterClient(channel); var reply = await client.SayHelloAsync (new HelloRequest { Name = "mycodebit.com" }); Console.WriteLine(reply.Message); Console.ReadKey(); }
Now lets run our client application.
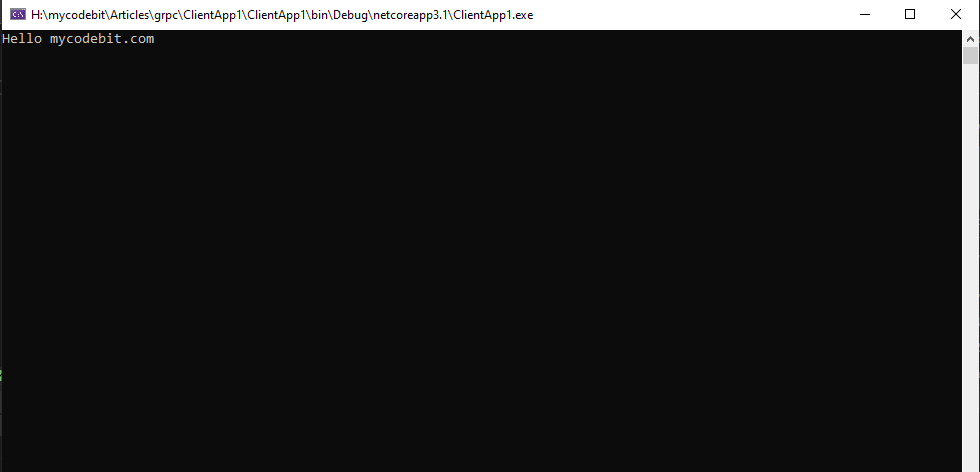
So we sent message from our client application and received the response from gRPC Applicaton.
We can build build microservices-based applications, native mobile applications, and Internet of Things (IoT) applications and take advantages of bi-directional streaming and binary messages formate for information exchange.
We don’t have to create controllers on server like RESTful Services in gRPC services or build HTTP requests on client side to use the services. We will go into more deep details in gRPC services.
Conclusion:
In this article, we have discussed and learned how to create gRPC Application in ASP.NET Core and How to send request from client to gRPC Application in ASP.NET Core. We will deep dive into gRPC in further article keep visiting us. If you have any suggestion to improve ourself or have any query to ask. Do not hesitate to contact us or you can comment below.