In this article, we are going to discuss How to Implement Multiple Interfaces Having Same Method Name in C#.
What is interface in C#?
In C#, Interfaces are like classes they can have methods properties, events, and indexers members. But interfaces only of signatures or declaration of methods. The implementation of these methods is done by classes that implement the interface implicitly or explicitly. C# allows us to implement multiple interfaces with the method names in one class which is derived by multiple interfaces.
To understand this let’s move to an example.
Example:
We have created two interfaces in this example and named “Student1” and “Student2”.
interface Student1 { // interface method void RollNo(); } interface Student2 { // interface method void RollNo(); }
Now let’s implement the methods into a class. We have implemented both methods separately.
public class DemoClass : Student1, Student2 { //Implemented both methods separately void Student1.RollNo() { Console.WriteLine("Student1 Roll No is 1."); } void Student2.RollNo() { Console.WriteLine("Student2 Roll No is 2."); } }
Lets have a complete example code to get a complete understanding.
using System; namespace multiple_interfaces_demo { interface Student1 { // interface method void RollNo(); } interface Student2 { // interface method void RollNo(); } public class DemoClass : Student1, Student2 { //Implemented both methods separately void Student1.RollNo() { Console.WriteLine("Student1 Roll No is 1."); } void Student2.RollNo() { Console.WriteLine("Student2 Roll No is 2."); } } internal class Program { static void Main(string[] args) { Student1 student1 = new DemoClass(); // calling method student1.RollNo(); Student2 student2 = new DemoClass(); // calling method student2.RollNo(); } } }
In this example, we have implemented two interfaces Student1 and Student2 with the same method name in a single derived class DemoClass. Now let’s run the code.
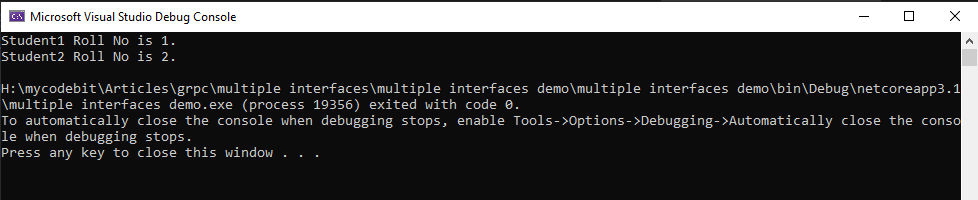
Here we have our output without any error. That’s how you can implement multiple interfaces with the same method name in one class.
Conclusion:
In this tutorial, we have learned How to Implement Multiple Interfaces Having the Same Method Name. If you have any suggestion to improve ourself or have any query to ask. Do not hesitate to contact us or you can comment below.